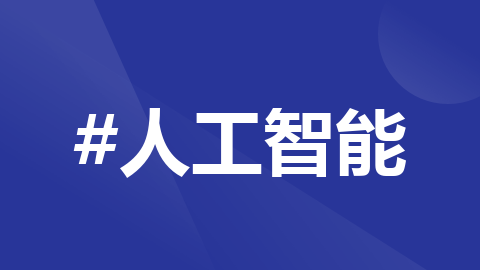
语音识别与语音合成库
是 Annyang.js 中的一个通配符,表示匹配任何语音输入中的文本,并将其作为参数传递给命令回调函数。匹配 “cats”,并将其作为参数传递给回调函数。回调函数可以使用这个参数来执行搜索操作。
·
截止目前已有的语音识别与语音合成库都比较老,建议直接使用web-speech-api自己封装。
web-speech-api必须在https环境使用或者在Chrome108版本使用(亲测高版本会直接限制语音使用),且必须能够连接外网。
Annyang.js库
*text
是 Annyang.js 中的一个通配符,表示匹配任何语音输入中的文本,并将其作为参数传递给命令回调函数。
例如,如果用户说 “search for cats”,则可以使用以下命令来捕获输入中的搜索词:
const commands = {
'search for *text': (text) => {
console.log('Search for:', text);
// 在这里执行搜索操作...
},
};
在这个例子中,*text
匹配 “cats”,并将其作为参数传递给回调函数。回调函数可以使用这个参数来执行搜索操作。
<!DOCTYPE html>
<html>
<head>
<title>Annyang Speech Recognition</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.4/css/all.min.css" crossorigin="anonymous" referrerpolicy="no-referrer" />
<style>
.microphone {
width: 18px;
height: 18px;
background-color: #00ff7f; /* 浅绿色 */
border-radius: 50%;
margin-bottom: 10px;
animation-name: pulse;
animation-duration: 1s;
animation-iteration-count: infinite;
text-align: center;
}
@keyframes pulse {
0% {
transform: scale(1);
box-shadow: 0 0 0 0 rgba(0, 255, 127, 0.7);
}
50% {
transform: scale(1.2);
box-shadow: 0 0 0 6px rgba(0, 255, 127, 0);
}
100% {
transform: scale(1);
box-shadow: 0 0 0 0 rgba(0, 255, 127, 0);
}
}
</style>
</head>
<body>
<input type="text" id="input" placeholder="说话后将回显到此处" />
<i id="microphone" onclick="controlPhone()" class="fas fa-microphone"></i>
<script src="https://cdnjs.cloudflare.com/ajax/libs/annyang/2.6.1/annyang.min.js"></script>
<script>
let starting = false;
// 初始化Annyang
if (annyang) {
var input = document.getElementById('input');
var microphone = document.getElementById('microphone');
// 定义语音识别命令
var commands = {
'*text': function (text) {
input.value = text;
}
};
// 添加语音识别命令
annyang.setLanguage('zh-CN');
annyang.addCommands(commands);
// annyang.start();
// 当语音识别开始时添加动效
// annyang.addCallback('start', function() {
// microphone.classList.add('microphone');
// });
// // 当语音识别结束时移除动效
// annyang.addCallback('end', function() {
// microphone.classList.remove('microphone');
// });
function controlPhone() {
starting = !starting;
if (starting) {
// 启动语音识别
annyang.start();
// 播放动画
microphone.classList.add('microphone');
} else {
annyang.abort();
microphone.classList.remove('microphone');
}
}
}
</script>
</body>
</html>
web speech API
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>语音识别Demo</title>
</head>
<body>
<h1>语音识别Demo</h1>
<button id="startButton">开始识别</button>
<button id="stopButton">停止识别</button>
<input type="text" id="textInput" placeholder="语音识别结果" />
<script>
class SpeechRecognitionLibrary {
constructor() {
this.recognition = new webkitSpeechRecognition();
this.recognition.lang = "zh-CN"; // 将语言设置为中文(简体中文)
this.recognition.continuous = true;
this.recognition.interimResults = false;
this.isListening = false;
// 识别结果回调函数
this.onResultCallback = null;
// 添加语音识别结果事件处理程序
this.recognition.onresult = (event) => {
// console.log(33, event);
const result = event.results[0][0].transcript;
console.log(1, result)
if (this.onResultCallback) {
this.onResultCallback(result);
}
};
}
startListening() {
if (!this.isListening) {
console.log(2);
this.recognition.start();
this.isListening = true;
}
}
stopListening() {
if (this.isListening) {
this.recognition.stop();
this.isListening = false;
}
}
onResult(callback) {
this.onResultCallback = callback;
}
}
// 示例用法
const speechRecognizer = new SpeechRecognitionLibrary();
speechRecognizer.onResult((result) => {
console.log("识别结果:", result);
});
speechRecognizer.onerror = (event) => {
console.error("语音识别错误:", event.error);
};
document.getElementById("startButton").addEventListener("click", () => {
speechRecognizer.startListening();
});
document.getElementById("stopButton").addEventListener("click", () => {
speechRecognizer.stopListening();
});
</script>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>中文语音识别示例</title>
</head>
<body>
<h1>中文语音识别示例</h1>
<button id="startButton">开始语音识别</button>
<p id="result"></p>
<script>
const startButton = document.getElementById('startButton');
const result = document.getElementById('result');
if ('webkitSpeechRecognition' in window) {
const recognition = new webkitSpeechRecognition();
recognition.lang = 'zh-CN'; // 设置识别语言为中文
startButton.addEventListener('click', () => {
recognition.start();
startButton.disabled = true;
startButton.textContent = '正在识别...';
});
recognition.onresult = (event) => {
const transcript = event.results[0][0].transcript;
result.textContent = '识别结果:' + transcript;
startButton.disabled = false;
startButton.textContent = '开始语音识别';
};
recognition.onend = () => {
startButton.disabled = false;
startButton.textContent = '开始语音识别';
};
} else {
result.textContent = '抱歉,你的浏览器不支持语音识别。';
startButton.disabled = true;
}
</script>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<title>中文语音识别示例</title>
</head>
<body>
<h1>中文语音识别示例</h1>
<button id="startButton">开始语音识别</button>
<p id="result"></p>
<script>
const startButton = document.getElementById("startButton");
const result = document.getElementById("result");
if ("webkitSpeechRecognition" in window) {
const recognition = new webkitSpeechRecognition();
recognition.lang = "zh-CN"; // 设置识别语言为中文
recognition.interimResults = true;
recognition.maxAlternatives = 5; // 获取多达5个备选结果
recognition.mode = "speech"; // 或 'audio',根据你的需求
startButton.addEventListener("click", () => {
recognition.start();
startButton.disabled = true;
startButton.textContent = "正在识别...";
});
recognition.onresult = (event) => {
console.log(1, )
const transcript = event.results[0][0].transcript;
result.textContent = "识别结果:" + transcript;
startButton.disabled = false;
startButton.textContent = "开始语音识别";
};
recognition.onerror = function (event) {
// 处理错误
console.error("发生错误:" + event.error);
};
recognition.onend = () => {
startButton.disabled = false;
console.log('识别结束')
startButton.textContent = "开始语音识别";
};
} else {
result.textContent = "抱歉,你的浏览器不支持语音识别。";
startButton.disabled = true;
}
</script>
</body>
</html>
更多推荐
所有评论(0)