conn_with_logging.py
#!/usr/bin/env python
from netmiko import Netmiko
from getpass import getpass
import logging
logging.basicConfig(filename='D:\\netmiko-develop\\netmiko-develop\\examples\\use_cases\\case11_logging\\test.log', level=logging.DEBUG)
logger = logging.getLogger("netmiko")
cisco1 = {
'host': '10.0.1.7',
'username': 'admin',
'password': getpass(),
'device_type': 'cisco_ios',
}
net_connect = Netmiko(**cisco1)
print(net_connect.find_prompt())
net_connect.disconnect()
终端显示是这样的
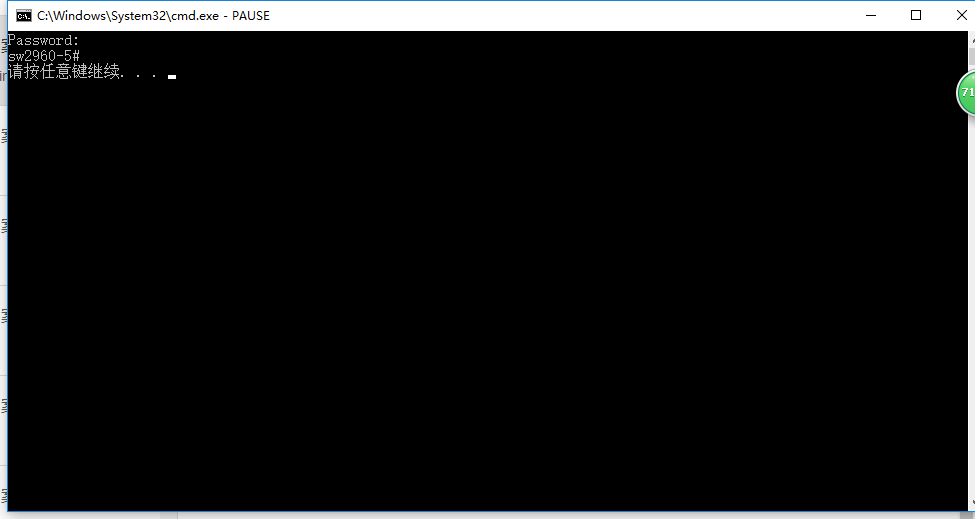
image.png
test.log日志记录是这样的
DEBUG:paramiko.transport:starting thread (client mode): 0x68c42b0L
DEBUG:paramiko.transport:Local version/idstring: SSH-2.0-paramiko_2.4.1
DEBUG:paramiko.transport:Remote version/idstring: SSH-1.99-Cisco-1.25
INFO:paramiko.transport:Connected (version 1.99, client Cisco-1.25)
DEBUG:paramiko.transport:kex algos:[u'diffie-hellman-group-exchange-sha1', u'diffie-hellman-group14-sha1', u'diffie-hellman-group1-sha1'] server key:[u'ssh-rsa'] client encrypt:[u'aes128-ctr', u'aes192-ctr', u'aes256-ctr', u'aes128-cbc', u'3des-cbc', u'aes192-cbc', u'aes256-cbc'] server encrypt:[u'aes128-ctr', u'aes192-ctr', u'aes256-ctr', u'aes128-cbc', u'3des-cbc', u'aes192-cbc', u'aes256-cbc'] client mac:[u'hmac-sha1', u'hmac-sha1-96'] server mac:[u'hmac-sha1', u'hmac-sha1-96'] client compress:[u'none'] server compress:[u'none'] client lang:[u''] server lang:[u''] kex follows?False
DEBUG:paramiko.transport:Kex agreed: diffie-hellman-group-exchange-sha1
DEBUG:paramiko.transport:HostKey agreed: ssh-rsa
DEBUG:paramiko.transport:Cipher agreed: aes128-ctr
DEBUG:paramiko.transport:MAC agreed: hmac-sha1
DEBUG:paramiko.transport:Compression agreed: none
DEBUG:paramiko.transport:Got server p (2048 bits)
DEBUG:paramiko.transport:kex engine KexGex specified hash_algo <built-in function openssl_sha1>
DEBUG:paramiko.transport:Switch to new keys ...
DEBUG:paramiko.transport:Adding ssh-rsa host key for 10.0.1.7: e15bc6df81cad6543167301464b1098b
DEBUG:paramiko.transport:userauth is OK
INFO:paramiko.transport:Authentication (password) successful!
DEBUG:paramiko.transport:[chan 0] Max packet in: 32768 bytes
DEBUG:paramiko.transport:[chan 0] Max packet out: 4096 bytes
DEBUG:paramiko.transport:Secsh channel 0 opened.
DEBUG:paramiko.transport:[chan 0] Sesch channel 0 request ok
DEBUG:paramiko.transport:[chan 0] Sesch channel 0 request ok
DEBUG:netmiko:read_channel:
sw2960-5#
DEBUG:netmiko:read_channel:
DEBUG:netmiko:read_channel:
DEBUG:netmiko:read_channel:
DEBUG:netmiko:write_channel:
DEBUG:netmiko:read_channel:
sw2960-5#
DEBUG:netmiko:read_channel:
DEBUG:netmiko:read_channel:
DEBUG:netmiko:In disable_paging
DEBUG:netmiko:Command: terminal length 0
DEBUG:netmiko:write_channel: terminal length 0
DEBUG:netmiko:Pattern is: sw2960\-5
DEBUG:netmiko:_read_channel_expect read_data: t
DEBUG:netmiko:_read_channel_expect read_data: erminal length 0
sw2960-5#
DEBUG:netmiko:Pattern found: sw2960\-5 terminal length 0
sw2960-5#
DEBUG:netmiko:terminal length 0
sw2960-5#
DEBUG:netmiko:Exiting disable_paging
DEBUG:netmiko:write_channel: terminal width 511
DEBUG:netmiko:Pattern is: sw2960\-5
DEBUG:netmiko:_read_channel_expect read_data: t
DEBUG:netmiko:_read_channel_expect read_data: erminal width 511
sw2960-5#
DEBUG:netmiko:Pattern found: sw2960\-5 terminal width 511
sw2960-5#
DEBUG:netmiko:read_channel:
DEBUG:netmiko:read_channel:
DEBUG:netmiko:write_channel:
DEBUG:netmiko:read_channel:
sw2960-5#
DEBUG:netmiko:read_channel:
DEBUG:netmiko:write_channel:
DEBUG:netmiko:read_channel:
sw2960-5#
DEBUG:netmiko:read_channel:
DEBUG:netmiko:read_channel:
DEBUG:netmiko:exit_config_mode:
DEBUG:netmiko:write_channel: exit
DEBUG:paramiko.transport:EOF in transport thread
write_channel.py
#!/usr/bin/env python
from netmiko import Netmiko
from getpass import getpass
import time
cisco1 = {
'host': '10.0.1.7',
'username': 'admin',
'password': getpass(),
'device_type': 'cisco_ios',
}
net_connect = Netmiko(**cisco1)
print(net_connect.find_prompt())
net_connect.write_channel("show ip int brief\n")
time.sleep(1)
output = net_connect.read_channel()
print(output)
net_connect.disconnect()
所有评论(0)