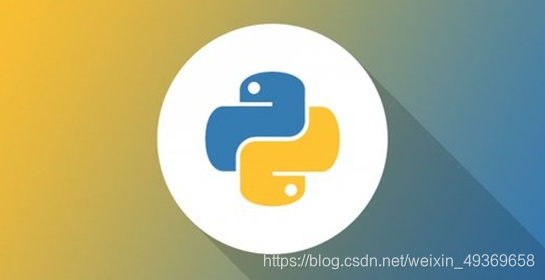
Python | 系统编程概述(一)
### 获取模块文档```python>>> import sys, os>>> len(dir(sys))89>>> len(dir(os))333>>> len(dir(os.path))55>>> dir(sys)['__breakpointhook__', '__displayhook__', '__
·
更多详情,请查看 www.houyuze.xyz
获取模块文档
>>> import sys, os
>>> len(dir(sys))
89
>>> len(dir(os))
333
>>> len(dir(os.path))
55
>>> dir(sys)
['__breakpointhook__', '__displayhook__', '__doc__', '__excepthook__', '__interactivehook__', '__loader__', '__name__', '__package__', '__spec__', '__stderr__', '__stdin__', '__stdout__', '_clear_type_cache', '_current_frames', '_debugmallocstats', '_framework', '_getframe', '_git', '_home', '_xoptions', 'abiflags', 'api_version', 'argv', 'base_exec_prefix', 'base_prefix', 'breakpointhook', 'builtin_module_names', 'byteorder', 'call_tracing', 'callstats', 'copyright', 'displayhook', 'dont_write_bytecode', 'exc_info', 'excepthook', 'exec_prefix', 'executable', 'exit', 'flags', 'float_info', 'float_repr_style', 'get_asyncgen_hooks', 'get_coroutine_origin_tracking_depth', 'get_coroutine_wrapper', 'getallocatedblocks', 'getcheckinterval', 'getdefaultencoding', 'getdlopenflags', 'getfilesystemencodeerrors', 'getfilesystemencoding', 'getprofile', 'getrecursionlimit', 'getrefcount', 'getsizeof', 'getswitchinterval', 'gettrace', 'hash_info', 'hexversion', 'implementation', 'int_info', 'intern', 'is_finalizing', 'maxsize', 'maxunicode', 'meta_path', 'modules', 'path', 'path_hooks', 'path_importer_cache', 'platform', 'prefix', 'ps1', 'ps2', 'set_asyncgen_hooks', 'set_coroutine_origin_tracking_depth', 'set_coroutine_wrapper', 'setcheckinterval', 'setdlopenflags', 'setprofile', 'setrecursionlimit', 'setswitchinterval', 'settrace', 'stderr', 'stdin', 'stdout', 'thread_info', 'version', 'version_info', 'warnoptions']
-
dir():返回一个列表,其中包含了带属性对象的所有属性的字符串名称
>>> sys.__doc__
"This module provides access to some objects used or maintained by the\ninterpreter and to functions that interact strongly with the interpreter.\n\nDynamic objects:\n\nargv -- command line arguments; argv[0] is the script pathname if known\npath -- module search path; path[0] is the script directory, else ''\nmodules -- dictionary of loaded modules\n\ndisplayhook -- called to show results in an interactive session\nexcepthook -- called to handle any uncaught exception other than SystemExit\n To customize printing in an interactive session or to install a custom\n top-level exception handler, assign other functions to replace these.\n\nstdin -- standard input file object; used by input()\nstdout -- standard output file object; used by print()\nstderr -- standard error object; used for error messages\n By assigning other file objects (or objects that behave like files)\n to these, it is possible to redirect all of the interpreter's I/O.\n\nlast_type -- type of last uncaught exception\nlast_value -- value of last uncaught exception\nlast_traceback -- traceback of last uncaught exception\n These three are only available in an interactive session after a\n traceback has been printed.\n\nStatic objects:\n\nbuiltin_module_names -- tuple of module names built into this interpreter\ncopyright -- copyright notice pertaining to this interpreter\nexec_prefix -- prefix used to find the machine-specific Python library\nexecutable -- absolute path of the executable binary of the Python interpreter\nfloat_info -- a struct sequence with information about the float implementation.\nfloat_repr_style -- string indicating the style of repr() output for floats\nhash_info -- a struct sequence with information about the hash algorithm.\nhexversion -- version information encoded as a single integer\nimplementation -- Python implementation information.\nint_info -- a struct sequence with information about the int implementation.\nmaxsize -- the largest supported length of containers.\nmaxunicode -- the value of the largest Unicode code point\nplatform -- platform identifier\nprefix -- prefix used to find the Python library\nthread_info -- a struct sequence with information about the thread implementation.\nversion -- the version of this interpreter as a string\nversion_info -- version information as a named tuple\n__stdin__ -- the original stdin; don't touch!\n__stdout__ -- the original stdout; don't touch!\n__stderr__ -- the original stderr; don't touch!\n__displayhook__ -- the original displayhook; don't touch!\n__excepthook__ -- the original excepthook; don't touch!\n\nFunctions:\n\ndisplayhook() -- print an object to the screen, and save it in builtins._\nexcepthook() -- print an exception and its traceback to sys.stderr\nexc_info() -- return thread-safe information about the current exception\nexit() -- exit the interpreter by raising SystemExit\ngetdlopenflags() -- returns flags to be used for dlopen() calls\ngetprofile() -- get the global profiling function\ngetrefcount() -- return the reference count for an object (plus one :-)\ngetrecursionlimit() -- return the max recursion depth for the interpreter\ngetsizeof() -- return the size of an object in bytes\ngettrace() -- get the global debug tracing function\nsetcheckinterval() -- control how often the interpreter checks for events\nsetdlopenflags() -- set the flags to be used for dlopen() calls\nsetprofile() -- set the global profiling function\nsetrecursionlimit() -- set the max recursion depth for the interpreter\nsettrace() -- set the global debug tracing function\n"
-
使用“模块.属性”的格式即可访问一个属性
>>> print(sys.__doc__)
This module provides access to some objects used or maintained by the
interpreter and to functions that interact strongly with the interpreter.
Dynamic objects:
argv -- command line arguments; argv[0] is the script pathname if known
path -- module search path; path[0] is the script directory, else ''
modules -- dictionary of loaded modules
…
-
sys.__doc__含有文件编制字符串(如\n, \t),使用print()即可解释换行符
>>> help(sys)
Help on built-in module sys:
NAME
sys
MODULE REFERENCE
https://docs.python.org/3.7/library/sys
The following documentation is automatically generated from the Python
source files. It may be incomplete, incorrect or include features that
are considered implementation detail and may vary between Python
implementations. When in doubt, consult the module reference at the
location listed above.
...
-
help()是PyDoc系统提供的接口之一,可将对象相关的文档(文档字符串和结构信息等)呈现为格式化之后的形式
一个自定义分页的脚本
"""
分隔字符串或文本文件并交互地进行分页
"""
def more(text, numlines=15):
lines = text.splitlines() # 效果类似split('\n'),不过没有在末尾加''
while lines:
chunk = lines[:numlines]
lines = lines[numlines:]
for line in chunk: print(line)
if lines and input('More?') not in ['y', 'Y']: break
if __name__ == '__main__':
import sys # 运行时在此操作,导入时不进行
more(open(sys.argv[1]).read(), 10) # 显示命令行里的文件的页面内容
split与splitlines的区别
>>> line = 'aaa\nbbb\nccc\n'
>>> line.split('\n')
['aaa', 'bbb', 'ccc', '']
>>> line.splitlines
<built-in method splitlines of str object at 0x7fc0f02215b0>
>>> line.splitlines()
['aaa', 'bbb', 'ccc']
字符串方法基础知识
>>> mystr = 'xxxSPAMxxx'
>>> mystr.find('SPAM') # 返回首个匹配的位置偏移
3
>>> mystr.find('b') # find()未找到时
-1
>>> mystr.find('A')
-1
>>> mystr = 'XXXaaXXXaXXXaaaaaXXX'
>>> mystr.replace('aa', 'SPAM') # 全局替换
'XXXSPAMXXXaXXXSPAMSPAMaXXX'
>>> print(mystr) # 字符串不可变
XXXaaXXXaXXXaaaaaXXX
>>> 'SPAM' in mystr # 字符串内容测试
False
>>> 'A' in mystr
False
>>> 'a' in mystr
True
>>> mystr = ' \n abc \n\t'
>>> mystr.strip() # 取出空白符
'abc'
>>> mystr
' \n abc \n\t'
>>> mystr.rstrip() # 右侧取出
' \n abc'
>>> mystr.lstrip() # 左侧取出
'abc \n\t'
>>> mystr = 'BEACHERhou'
>>> mystr.lower() # 大小写转换
'beacherhou'
>>> mystr.upper()
'BEACHERHOU'
>>> mystr.isalpha() # 内容测试
True
>>> mystr.isdigit()
False
>>> mystr = 'aaa,bbb,ccc'
>>> mystr.split(',') # 分割为子字符串组成的列表
['aaa', 'bbb', 'ccc']
>>> mystr = 'a b\nc\nd' # 默认分割符:泛空格符
>>> mystr.split()
['a', 'b', 'c', 'd']
>>> mystr = 'a \nb\nc\n d'
>>> mystr.split()
['a', 'b', 'c', 'd']
>>> delim = 'NI'
>>> delim.join(['aaa', 'bbb', 'ccc']) # 连接子字符串列表
'aaaNIbbbNIccc'
>>> ' '.join(['aaa', 'bbb', 'ccc']) # 在其间添加空格符
'aaa bbb ccc'
>>> chars = list('beacher') # 转换为字符组成的列表
>>> chars
['b', 'e', 'a', 'c', 'h', 'e', 'r']
>>> chars.append('!')
>>> ''.join(chars) # 生成字符串:分割符为空
'beacher!'
>>> mystr = 'xxaaxxaaxx'
>>> 'SPAM'.join(mystr.split('aa')) # 效果等同于str.replace
'xxSPAMxxSPAMxx'
>>> int('42') # 字符串转换为整型
42
>>> int('42'), eval('42') # 整型转换为字符串
(42, 42)
>>> str(42), repr(42)
('42', '42')
>>> ('%d' % 12), '{:d}'.format(42) # 分别借助格式化表达式和方法
('12', '42')
>>> '42' + str(1), int('42') + 1 # 分别为连接和加法
('421', 43)
文件操作基础知识
open('file').read() # 将整个文件读取为字符串
open('file').read(N) # 将后面N个字节读取为字符串
open('file').readlines() # 将整个文件读取为单行字符串组成的列表
open('file').readline() # 跨过'\n'读取下一行
>>> file = open('spam.txt', 'w') # 创建文件
>>> file.write(('spam' * 5) + '\n') # 写入文本:返回所写入的#个文本
21
>>> file.close()
>>> file = open('spam.txt') # 或者用 open('spam.txt').read()
>>> file.read()
'spamspamspamspamspam\n'
更多推荐
所有评论(0)